CoherenceBridge
The Bridge establishes a connection between your scene and the coherence Replication Server. It makes sure all networked entities stay in sync.
When you place a GameObject in your scene, the Bridge detects it and makes sure all the synchronization can be done via the CoherenceSync
component.

At runtime, you can inspect which Entities the Bridge is currently tracking.
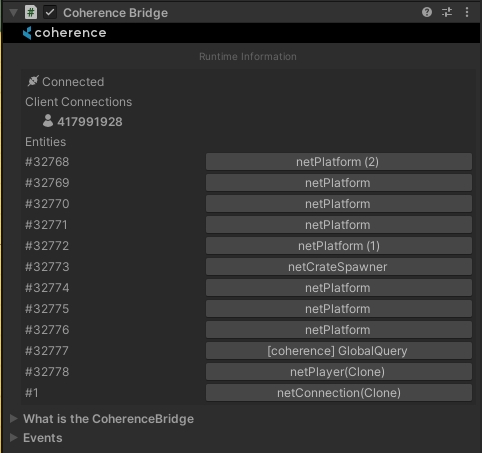
A Bridge is associated with the scene it's instantiated on, and keeps track of Entities that are part of that scene. This also allows for multiple connections at the same time coming from the game or within the Unity Editor.
You can use CoherenceBridgeStore.TryGetBridge to get a CoherenceBridge associated with a scene:
if (CoherenceBridgeStore.TryGetBridge(gameObject.scene, out var bridge))
{
Debug.Log("Bridge found");
}
Unity Events
The CoherenceBridge offers a couple of Unity Events in its inspector where you can hook your custom game logic:
OnLiveQuerySynced
This event is invoked when the Replication Server state has been fully synchronized, it is fired after OnConnected.
For example, if you connect to a ongoing game that has five players connected, when this event is fired all the entities and information of all the other players will already be synchronized and available to be polled.
OnConnected
This event is invoked the moment you stablish a connection with the Replication Server, but before any synchronization has happened.
Following the previous example, if you connect to an ongoing game that has five players connected, when this event is fired, you won't have any entities or information available about those five players.
OnDisconnected
This event is invoked when you disconnect from a Replication Server. In the parameters of the event you will be given a ConnectionCloseReason value that will explain why the disconnection happened.
OnConnectionError
This event is invoked when you attempt to connect to a Replication Server, but the connection fails, you will be returned a ConnectionException with information about the error.
Client Connections
The Client Connections system allows you to keep track of how many users are connected and uniquely identify them, as well as easily send server-wide messages.
You can read more about the Client Connections system here.
Connecting to Worlds/Rooms from coherence Cloud
If you have a developer account and have logged in, you can connect to Worlds or Rooms hosted in the coherence Cloud. After you fetch a valid World or Room using cloud services, you can use the JoinWorld or JoinRoom methods to easily connect your Client.
You can read more about the coherence Cloud Service here.
Last updated
Was this helpful?